Objectives
- Set-up a cartesian projection for the Cross section
- Configure the axis
- Load a netCDF file, and understand the information needed by Magics.
- Apply a shading
- Position the legend box
- Add a text.
You will need to download
Setting of the cartesian projection
We want to show our Cross section in the following cartesian system:
- The vertical coordinate system is a logarithmic axis from 1000 hPa to 200 hPa.
- The horizontal coordinate system is a geoline axis from [50oN, 90oW] to [30oN, 60oW]
Have a look at the subpage documentation to learn how to setup a cartesian projection .
We just have to add 2 axis (1 vertical, 1 horizontal ) to materialise it on the plot. For backward compatibility, we have only one maxis object, the orientation is defined using the parameter axis_orientation.
from Magics.macro import *
#setting the output
output = output(
output_formats = ['png'],
output_name = "xsect_step1",
output_name_first_page_number = "off"
)
# Setting the cartesian view
projection = mmap(
subpage_map_projection='cartesian',
subpage_x_axis_type='geoline',
subpage_y_axis_type='logarithmic',
subpage_x_min_latitude=50.,
subpage_x_max_latitude=30.,
subpage_x_min_longitude=-90.,
subpage_x_max_longitude=-60.,
subpage_y_min=1020.,
subpage_y_max=200.,
)
# Vertical axis
vertical = maxis(
axis_orientation='vertical',
)
# Horizontal axis
horizontal = maxis(
axis_orientation='horizontal',
)
plot(output, projection, horizontal, vertical)
Adjusting the axis
By specialising the axis, you can improve your axis visualisation.
Have a look at the Axis Documentation to browse the possibilities.
Now, try to improve the readability of the line by specialising the horizontal axis.
from Magics.macro import *
#setting the output
output = output(
output_formats = ['png'],
output_name = "xsect_step2",
output_name_first_page_number = "off"
)
# Setting the cartesian view
projection = mmap(
subpage_map_projection='cartesian',
subpage_x_axis_type='geoline',
subpage_y_axis_type='logarithmic',
subpage_x_min_latitude=50.,
subpage_x_max_latitude=30.,
subpage_x_min_longitude=-90.,
subpage_x_max_longitude=-60.,
subpage_y_min=1020.,
subpage_y_max=200.,
)
# Vertical axis
vertical = maxis(
axis_orientation='vertical',
axis_grid='on',
axis_type='logarithmic',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid_colour='charcoal',
axis_grid_line_style='dash',
axis_title='on',
axis_title_text='Pressure',
axis_title_height=0.6,
)
# Horizontal axis
horizontal = maxis(
axis_orientation='horizontal',
axis_type='geoline',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid='on',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_line_style='dash',
)
plot(output, projection, horizontal, vertical)
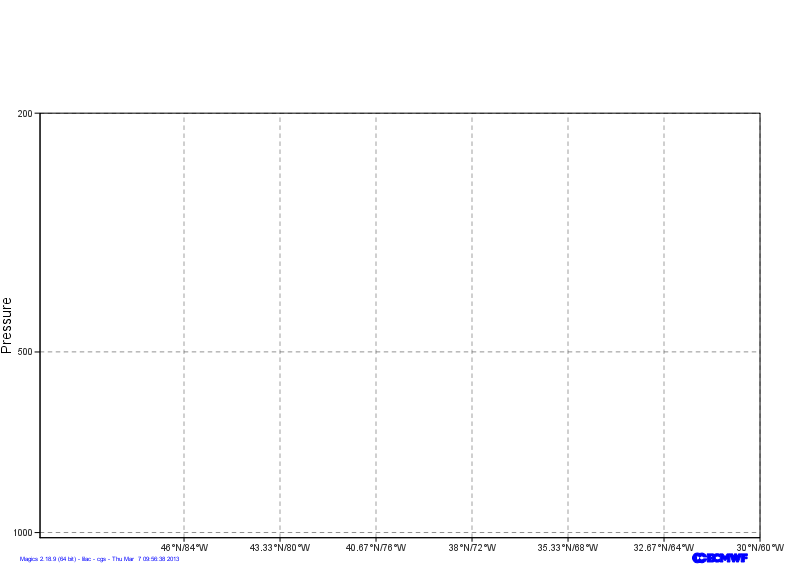
Loading the netCDF data
In this exercise, we want to visualise a matrix stored in a netCDF file. netCDF is a very generic format, and can contain a lot of data. We will need to set up some information in order to explain to Magics which variable to plot, and how to interpret it.
The mnetcdf data action comes with a parameter list that can be found in the netCDF Input Page.
But first, let's see what is inside our netCDF Data
netCDF section {
dimensions:
levels = 85 ;
longitude = 144 ;
latitude = 144 ;
p15220121030000000000001_1 = 2 ;
p15220121030000000000001_2 = 144 ;
orography_x_values = 144 ;
orography_y1_values = 144 ;
orography_y2_values = 144 ;
variables:
double levels(levels) ;
double longitude(longitude) ;
double latitude(latitude) ;
double p13820121030000000000001(levels, longitude) ;
We want to display the variable p13820121030000000000001, and inform Magics that the dimensions of the matrix are described in the 2 variables levels and longitude.
The range of the vorticity values is quite small, then we would like to apply a scaling factor of 100000.
We will then just apply a basic contouring.
from Magics.macro import *
#setting the output
output = output(
output_formats = ['png'],
output_name = "xsect_step3",
output_name_first_page_number = "off"
)
# Setting the cartesian view
projection = mmap(
subpage_map_projection='cartesian',
subpage_x_axis_type='geoline',
subpage_y_axis_type='logarithmic',
subpage_x_min_latitude=50.,
subpage_x_max_latitude=30.,
subpage_x_min_longitude=-90.,
subpage_x_max_longitude=-60.,
subpage_y_min=1020.,
subpage_y_max=200.,
)
# Vertical axis
vertical = maxis(
axis_orientation='vertical',
axis_grid='on',
axis_type='logarithmic',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_reference_line_style='solid',
axis_grid_reference_thickness=1,
axis_grid_line_style='dash',
axis_title='on',
axis_title_text='Pressure',
axis_title_height=0.6,
)
# Horizontal axis
horizontal = maxis(
axis_orientation='horizontal',
axis_type='geoline',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid='on',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_line_style='dash',
)
# Definition of the netCDF data and interpretation
data = mnetcdf(netcdf_filename = "section.nc",
netcdf_value_variable = "p13820121030000000000001",
netcdf_field_scaling_factor = 100000.,
netcdf_y_variable = "levels",
netcdf_x_variable = "longitude",
netcdf_x_auxiliary_variable = "latitude"
)
contour = mcont()
plot(output, projection, horizontal, vertical, data, contour)
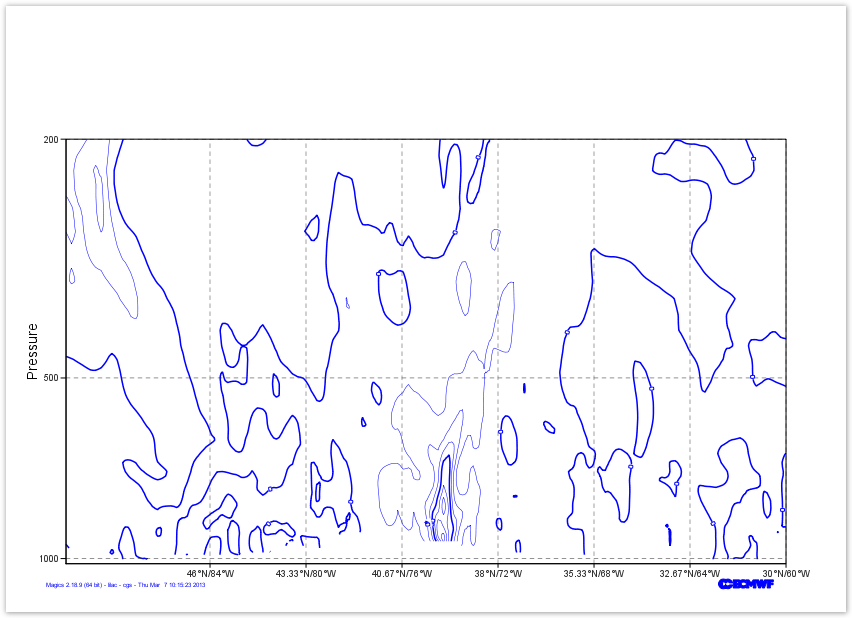
Creating a nice shading
Now, we just create a nice polygon shading.
The list of levels we want to use is : [-200., -100., -75., -50., -30., -20., -15., -13., -11., -9., -7., -5., -3., -1., 1., 3., 5., 7., 9., 11., 13., 15., 20., 30., 50., 75., 100., 200].
and the list of colours is : ["rgb(0,0,0.3)", "rgb(0,0,0.5)", "rgb(0,0,0.7)", "rgb(0,0,0.9)", "rgb(0,0.15,1)", "rgb(0,0.3,1)", "rgb(0,0.45,1)", "rgb(0,0.6,1)", "rgb(0,0.75,1)", "rgb(0,0.85,1)", "rgb(0.2,0.95,1)", "rgb(0.45,1,1)", "rgb(0.75,1,1)", "none", "rgb(1,1,0)","rgb(1,0.9,0)", "rgb(1,0.8,0)", "rgb(1,0.7,0)", "rgb(1,0.6,0)", "rgb(1,0.5,0)", "rgb(1,0.4,0)", "rgb(1,0.3,0)", "rgb(1,0.15,0)", "rgb(0.9,0,0)", "rgb(0.7,0,0)", "rgb(0.5,0,0)", "rgb(0.3,0,0)"],
Do not forget to turn the legend on ...
You could quickly check Contour Documentation
from Magics.macro import *
#setting the output
output = output(
output_formats = ['png'],
output_name = "xsect_step3",
output_name_first_page_number = "off"
)
# Setting the cartesian view
projection = mmap(
subpage_map_projection='cartesian',
subpage_x_axis_type='geoline',
subpage_y_axis_type='logarithmic',
subpage_x_min_latitude=50.,
subpage_x_max_latitude=30.,
subpage_x_min_longitude=-90.,
subpage_x_max_longitude=-60.,
subpage_y_min=1020.,
subpage_y_max=200.,
)
# Vertical axis
vertical = maxis(
axis_orientation='vertical',
axis_grid='on',
axis_type='logarithmic',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_reference_line_style='solid',
axis_grid_reference_thickness=1,
axis_grid_line_style='dash',
axis_title='on',
axis_title_text='Pressure',
axis_title_height=0.6,
)
# Horizontal axis
horizontal = maxis(
axis_orientation='horizontal',
axis_type='geoline',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid='on',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_line_style='dash',
)
# Definition of the netCDF data and interpretation
data = mnetcdf(netcdf_filename = "section.nc",
netcdf_value_variable = "p13820121030000000000001",
netcdf_field_scaling_factor = 100000.,
netcdf_y_variable = "levels",
netcdf_x_variable = "longitude",
netcdf_x_auxiliary_variable = "latitude"
)
contour = mcont(contour_highlight= "off",
contour_hilo= "off",
contour_label= "off",
legend='on',
contour_level_list= [-200., -100., -75., -50., -30., -20.,
-15., -13., -11., -9., -7., -5., -3., -1., 1., 3., 5.,
7., 9., 11., 13., 15., 20., 30., 50., 75., 100., 200],
contour_level_selection_type= "level_list",
contour_shade= "on",
contour_shade_colour_list= ["rgb(0,0,0.3)", "rgb(0,0,0.5)",
"rgb(0,0,0.7)", "rgb(0,0,0.9)", "rgb(0,0.15,1)",
"rgb(0,0.3,1)", "rgb(0,0.45,1)", "rgb(0,0.6,1)",
"rgb(0,0.75,1)", "rgb(0,0.85,1)", "rgb(0.2,0.95,1)",
"rgb(0.45,1,1)", "rgb(0.75,1,1)", "none", "rgb(1,1,0)",
"rgb(1,0.9,0)", "rgb(1,0.8,0)", "rgb(1,0.7,0)",
"rgb(1,0.6,0)", "rgb(1,0.5,0)", "rgb(1,0.4,0)",
"rgb(1,0.3,0)", "rgb(1,0.15,0)", "rgb(0.9,0,0)",
"rgb(0.7,0,0)", "rgb(0.5,0,0)", "rgb(0.3,0,0)"],
contour_shade_colour_method= "list",
contour_shade_method= "area_fill")
plot(output, projection, horizontal, vertical, data, contour)
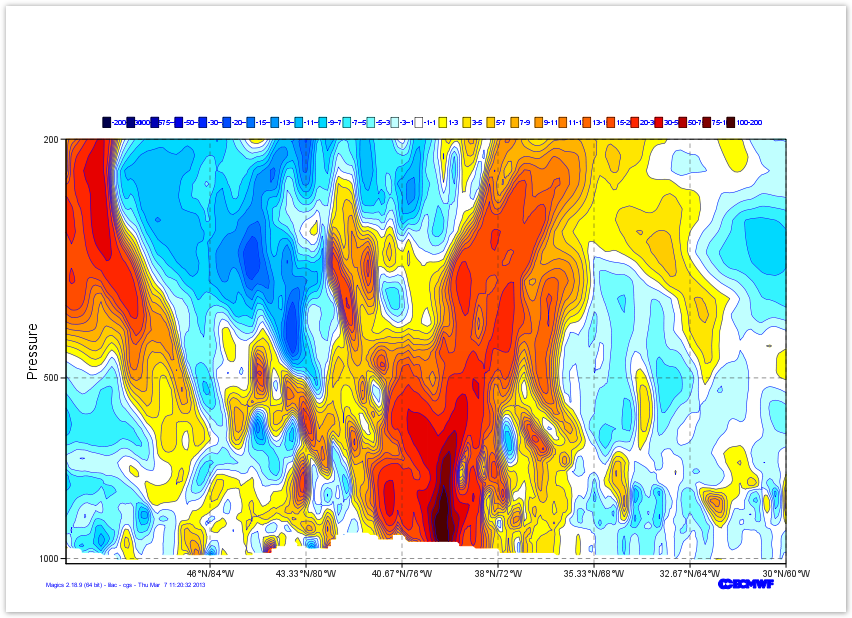
Position the legend
By default, the legend is is positioned at the top of the plot. In this exercise we want to put see our legend on a column mode on the right of the plot.
The Information about the positional mode can be found in the Legend Documentation
from Magics.macro import *
#setting the output
output = output(
output_formats = ['png'],
output_name = "xsect_step5",
output_name_first_page_number = "off"
)
# Setting the cartesian view
projection = mmap(
subpage_map_projection='cartesian',
subpage_x_axis_type='geoline',
subpage_y_axis_type='logarithmic',
subpage_x_min_latitude=50.,
subpage_x_max_latitude=30.,
subpage_x_min_longitude=-90.,
subpage_x_max_longitude=-60.,
subpage_y_min=1020.,
subpage_y_max=200.,
)
# Vertical axis
vertical = maxis(
axis_orientation='vertical',
axis_grid='on',
axis_type='logarithmic',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_reference_line_style='solid',
axis_grid_reference_thickness=1,
axis_grid_line_style='dash',
axis_title='on',
axis_title_text='Pressure',
axis_title_height=0.6,
)
# Horizontal axis
horizontal = maxis(
axis_orientation='horizontal',
axis_type='geoline',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid='on',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_line_style='dash',
)
# Definition of the netCDF data and interpretation
data = mnetcdf(netcdf_filename = "section.nc",
netcdf_value_variable = "p13820121030000000000001",
netcdf_field_scaling_factor = 100000.,
netcdf_y_variable = "levels",
netcdf_x_variable = "longitude",
netcdf_x_auxiliary_variable = "latitude"
)
contour = mcont(contour_highlight= "off",
contour_hilo= "off",
contour_label= "off",
legend='on',
contour_level_list= [-200., -100., -75., -50., -30., -20.,
-15., -13., -11., -9., -7., -5., -3., -1., 1., 3., 5.,
7., 9., 11., 13., 15., 20., 30., 50., 75., 100., 200],
contour_level_selection_type= "level_list",
contour_shade= "on",
contour_shade_colour_list= ["rgb(0,0,0.3)", "rgb(0,0,0.5)",
"rgb(0,0,0.7)", "rgb(0,0,0.9)", "rgb(0,0.15,1)",
"rgb(0,0.3,1)", "rgb(0,0.45,1)", "rgb(0,0.6,1)",
"rgb(0,0.75,1)", "rgb(0,0.85,1)", "rgb(0.2,0.95,1)",
"rgb(0.45,1,1)", "rgb(0.75,1,1)", "none", "rgb(1,1,0)",
"rgb(1,0.9,0)", "rgb(1,0.8,0)", "rgb(1,0.7,0)",
"rgb(1,0.6,0)", "rgb(1,0.5,0)", "rgb(1,0.4,0)",
"rgb(1,0.3,0)", "rgb(1,0.15,0)", "rgb(0.9,0,0)",
"rgb(0.7,0,0)", "rgb(0.5,0,0)", "rgb(0.3,0,0)"],
contour_shade_colour_method= "list",
contour_shade_method= "area_fill")
legend = mlegend(legend='on',
legend_display_type='continuous',
legend_text_colour='charcoal',
legend_text_font_size=0.4,
legend_box_mode = "positional",
legend_box_x_position = 27.00,
legend_box_y_position = 3.00,
legend_box_x_length = 2.00,
legend_box_y_length = 13.00,
legend_box_blanking = "on",
legend_border = "on",
legend_border_colour='charcoal')
plot(output, projection, horizontal, vertical, data, contour, legend)
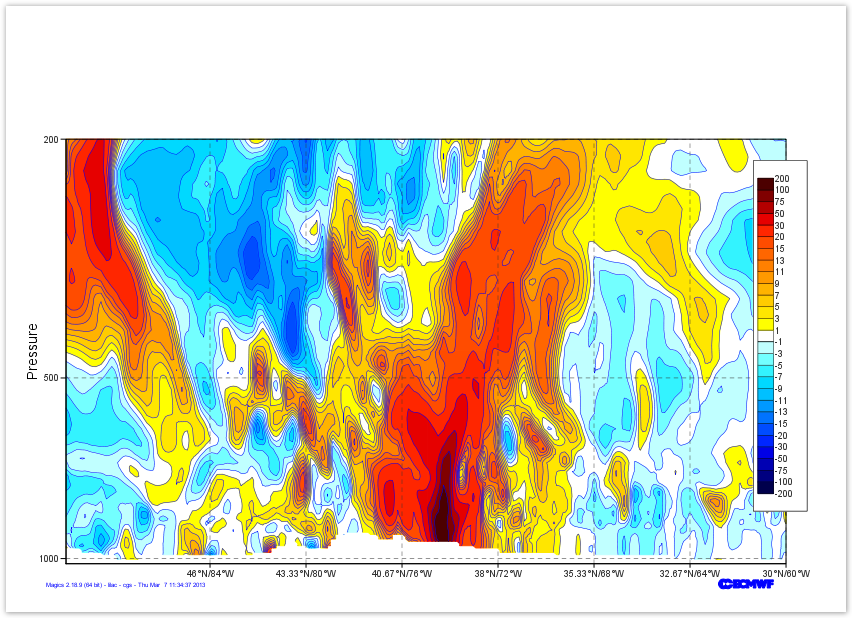
add a Title
Last thing to do here .. We add a title to the top..
Here we will use a combination of User text and automatic text ( read from the netCDF global attribute title)
To get the automatic title, you have to use the following tag <magics_title/>
Check the Text Plotting Page for more information
from Magics.macro import *
#setting the output
output = output(
output_formats = ['png'],
output_name = "xsect_step6",
output_name_first_page_number = "off"
)
# Setting the cartesian view
projection = mmap(
subpage_map_projection='cartesian',
subpage_x_axis_type='geoline',
subpage_y_axis_type='logarithmic',
subpage_x_min_latitude=50.,
subpage_x_max_latitude=30.,
subpage_x_min_longitude=-90.,
subpage_x_max_longitude=-60.,
subpage_y_min=1020.,
subpage_y_max=200.,
)
# Vertical axis
vertical = maxis(
axis_orientation='vertical',
axis_grid='on',
axis_type='logarithmic',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_reference_line_style='solid',
axis_grid_reference_thickness=1,
axis_grid_line_style='dash',
axis_title='on',
axis_title_text='Pressure',
axis_title_height=0.6,
)
# Horizontal axis
horizontal = maxis(
axis_orientation='horizontal',
axis_type='geoline',
axis_tick_label_height=0.4,
axis_tick_label_colour='charcoal',
axis_grid='on',
axis_grid_colour='charcoal',
axis_grid_thickness=1,
axis_grid_line_style='dash',
)
# Definition of the netCDF data and interpretation
data = mnetcdf(netcdf_filename = "section.nc",
netcdf_value_variable = "p13820121030000000000001",
netcdf_field_scaling_factor = 100000.,
netcdf_y_variable = "levels",
netcdf_x_variable = "longitude",
netcdf_x_auxiliary_variable = "latitude"
)
contour = mcont(contour_highlight= "off",
contour_hilo= "off",
contour_label= "off",
legend='on',
contour_level_list= [-200., -100., -75., -50., -30., -20.,
-15., -13., -11., -9., -7., -5., -3., -1., 1., 3., 5.,
7., 9., 11., 13., 15., 20., 30., 50., 75., 100., 200],
contour_level_selection_type= "level_list",
contour_shade= "on",
contour_shade_colour_list= ["rgb(0,0,0.3)", "rgb(0,0,0.5)",
"rgb(0,0,0.7)", "rgb(0,0,0.9)", "rgb(0,0.15,1)",
"rgb(0,0.3,1)", "rgb(0,0.45,1)", "rgb(0,0.6,1)",
"rgb(0,0.75,1)", "rgb(0,0.85,1)", "rgb(0.2,0.95,1)",
"rgb(0.45,1,1)", "rgb(0.75,1,1)", "none", "rgb(1,1,0)",
"rgb(1,0.9,0)", "rgb(1,0.8,0)", "rgb(1,0.7,0)",
"rgb(1,0.6,0)", "rgb(1,0.5,0)", "rgb(1,0.4,0)",
"rgb(1,0.3,0)", "rgb(1,0.15,0)", "rgb(0.9,0,0)",
"rgb(0.7,0,0)", "rgb(0.5,0,0)", "rgb(0.3,0,0)"],
contour_shade_colour_method= "list",
contour_shade_method= "area_fill")
legend = mlegend(legend='on',
legend_display_type='continuous',
legend_text_colour='charcoal',
legend_text_font_size=0.4,
legend_box_mode = "positional",
legend_box_x_position = 27.00,
legend_box_y_position = 3.00,
legend_box_x_length = 2.00,
legend_box_y_length = 13.00,
legend_box_blanking = "on",
legend_border = "on",
legend_border_colour='charcoal')
title = mtext(
text_lines= ['Example of Xsection to demonstrate the use of netCDF data...',
'<magics_title/>'],
text_html= 'true',
text_justification= 'left',
text_font_size= 0.8,
text_colour= 'charcoal',
)
plot(output, projection, horizontal, vertical, data, contour, legend, title)
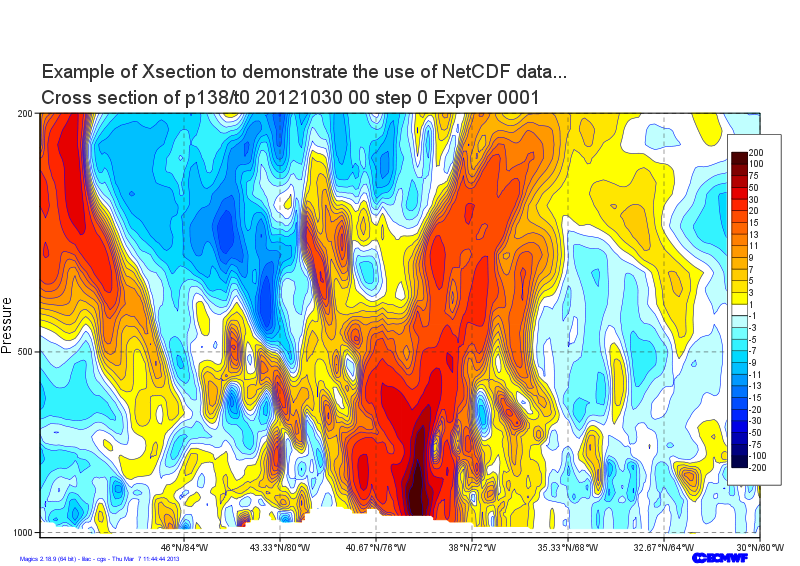
Go to the next step...
Go back to the main page ...
1 Comment
Yonghui Weng
I have installed Magics in my MacBook Pro with macOS 10.13.6, and encounter segmentation fault when run this example. Detail log are:
where Thread 8 crashed. Any idea? Thanks!